Creating meetings with your colleagues become a real-life must-do activity in our day to day life and also, A few things you think will be straightforward really wind up getting extremely mind-boggling.
Google realizes this all too well, which is the reason it as of late revealed a brand new Google Calendar and a lot of clever features to go with it.
I’ve started my internship about a month ago and I’m sailing through a busy windy climate these days, Got plenty of meetings on my plate with the management and Project leaders. But the good thing is that all the staff’s got this nice habit of creating a Calendar Meeting with the particular person so it’s unlikely to ignore or forget the time, place, what this meeting is for, how many people are participating in and a lot more. I never used Google Calendar before but now I feel like “Why the hell did Not!”
Although creating meetings a good thing, I’m too lazy to create 2,3 meetings on a day because I have to go through the same steps all over again.
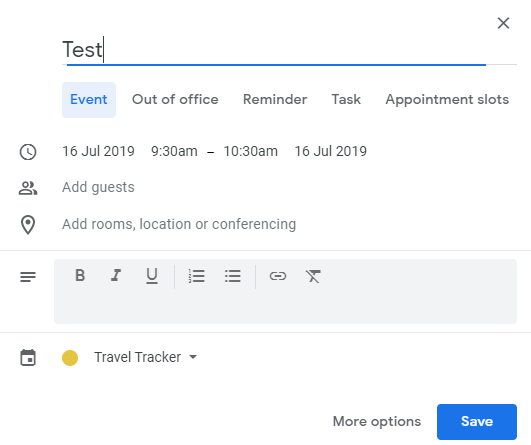
Alright, To kick things off lets head Google Developers Console and create a new project. I named mine as CalendarAPI. Then go to the dashboard and search for Calendar API in the search bar in order to enable the Calendar API for your project.
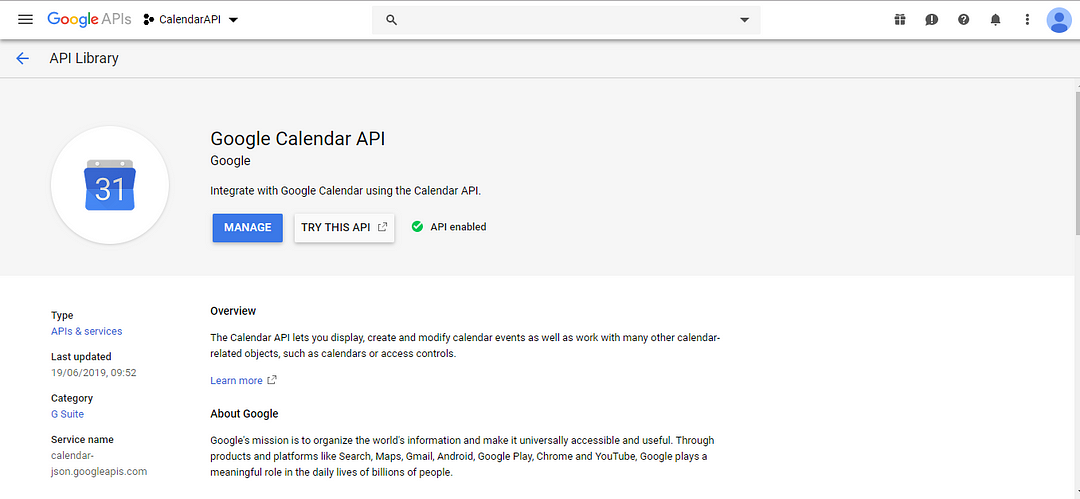
In my case it displays ‘Manage’ but if you’re enabling it for the first time you’ll see a button Enable instead of ‘Manage’
The first step is done, now we need credentials to access the API so click on Add Credentials on the next screen you see after enabling the API.
So the first thing you have to do after moving on to credentials section is go to the ‘OAuth Consent Screen’ tab, This one’s a bit different from API key, in fact, it’s safer and secure and it actually requires the user to give their consent before generating an actual token so that’s the main idea behind it. So let’s give it an application name so this will be visible to all the users who are using your application.
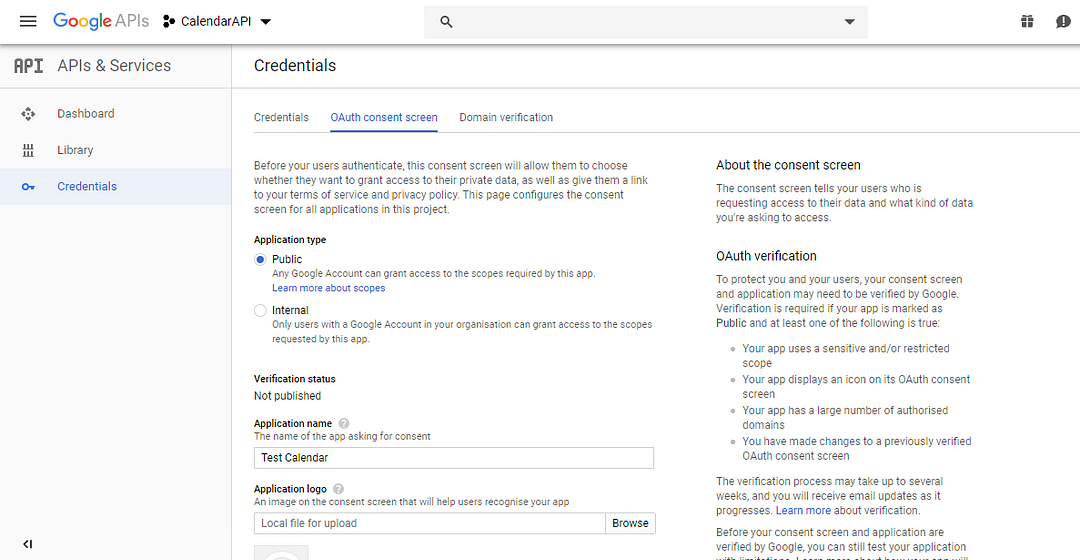
You won’t see the first “Application Type” option unless you’re using a domain-based email for your application. (@gmail.com is all fine) I named my application as Test Calendar and save it.
Now I’m gonna create an OAuth client ID as you can see the second option in below dropdown menu.
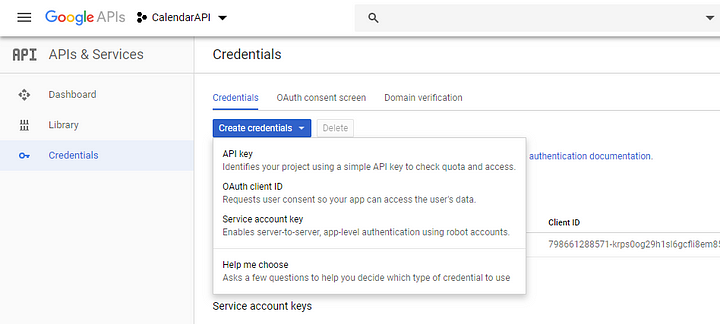
Then It’ll ask you the application type and select Other hence I don’t have any preference at the moment because I’ll be only using this to write some code to access my Calendar.
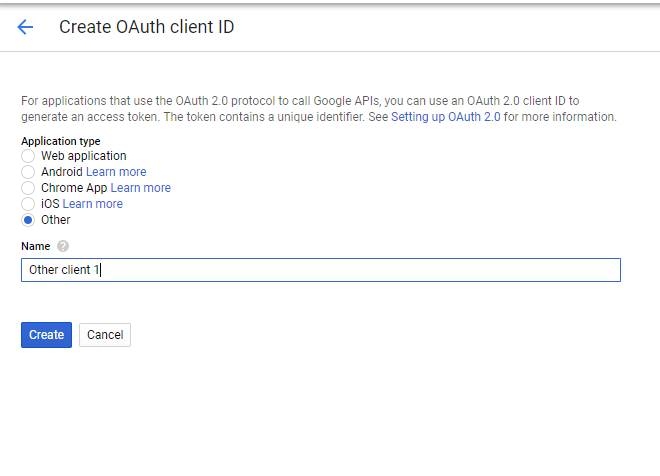
So this will generate your Client ID and Client Secret Keys but we want them as in a JSON file so move into that download button and download the JSON file with those credentials.
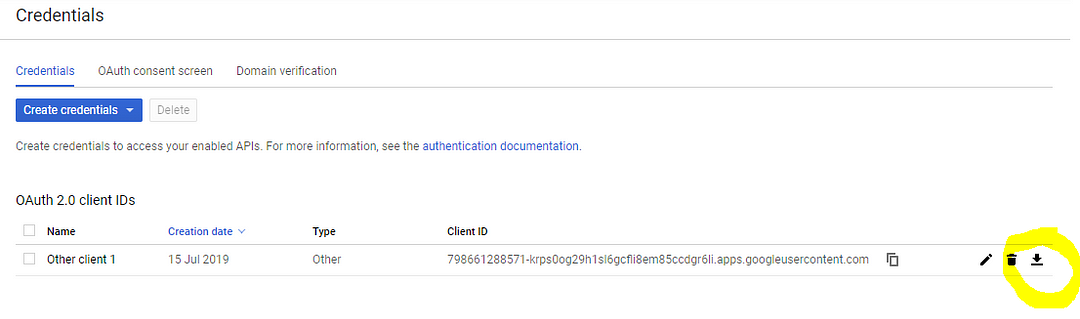
Find the file and rename it to “client_secret.json”. Now all the boring part is over and let’s do some coding! :)
So, the first thing that we’re going to do is importing essential libraries for our project.
from apiclient.discovery import build
from google_auth_oauthlib.flow import InstalledAppFlow
PS: If you haven't installed the Google API Python client API simply run the below pip command on your terminal.
pip install google-api python-client
And now what I have to do is I have to specify some scope for our project. That means which permissions I’m getting from the user, Click here to read more about SCOPE in Google Calendar API.
scopes = ['https://www.googleapis.com/auth/calendar']
Next thing is to create a flow:
flow = InstalledAppFlow.from_client_secrets_file("client_secret.json", scopes=scopes)
credentials = flow.run_console()
If you’re using the Jupyter notebook, Try Executing the above code and it will redirect you to your browser which asks you to select or login your Gmail account and it’ll generate this one time token.
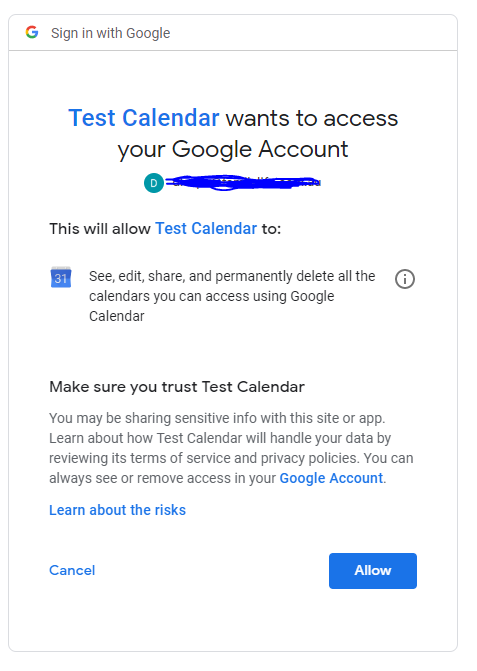
Doing this every time needs you run the app needs some anger management therapy yeah I know it :), So we can save these credentials in a Pickle file in python. Pickle is cable of saving any kind of python object inside the Pickle file.
pickle.dump(credentials, open("token.pkl", "wb"))
credentials = pickle.load(open("token.pkl", "rb"))
wb: Write Binary Mode
rb: Read Binary Mode
Now create a service object for our API using the build function from apiclient.discovery module we’ve imported earlier.
service = build(“calendar”, “v3”, credentials=credentials)
- Make sure you’re using version 3 since the second parameter is the version.
So now I’m ready to access my Calendar.
result = service.calendarList().list().execute()
This will display you all the calendars that you have in your Google Calendar because eventually, you’ll have more than one calendar if you’re syncing all the Birthdays of your contacts, Public holidays in your country…etc.
try running this in our command line/terminal
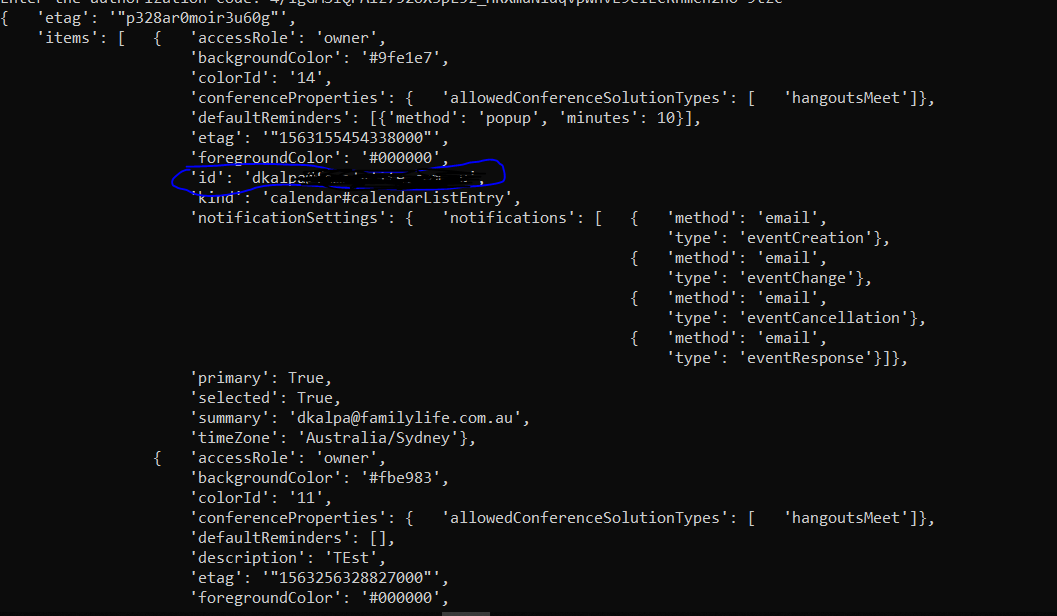
This will display all the calendars that you have in your Google Calendar. Check out the below screenshot if you’re still confusing about “What calendars? I have only one calendar! What is this man talking about?”
Chill dude, See this:
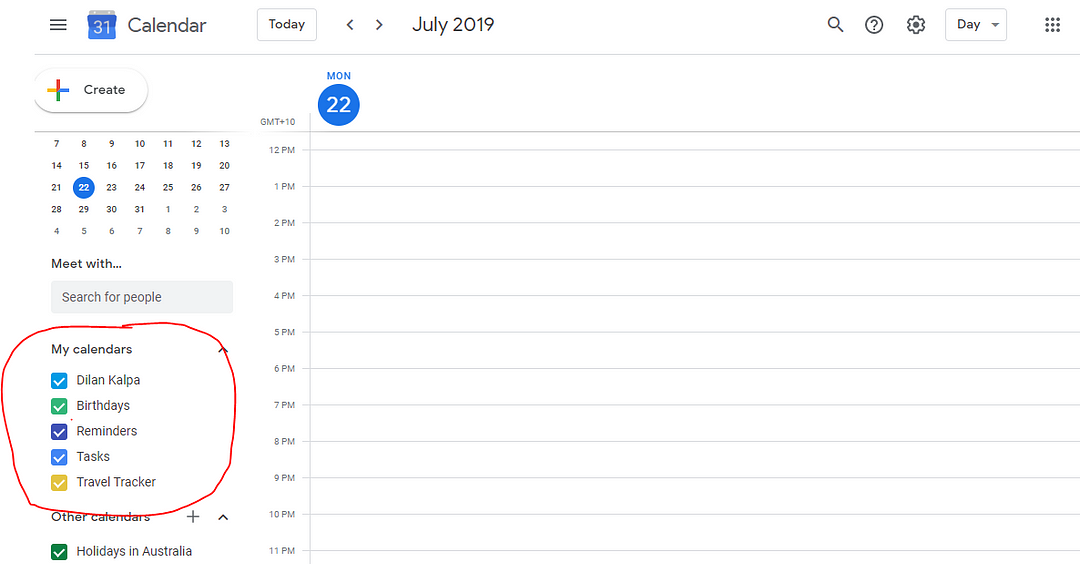
Ahh, that! Yeah, that.
Ok so, I’m only interested in my Primary Calendar which is ‘Dilan Kalpa’ on this list so I’ll grab my Calendar Id (See Commandline Screenshot rounded in blue color) and will store it in calendar_id variable
calendar_id = result['items'][0]['id']
Alrighty! Let’s see my events!
result = service.events().list(calendarId=calendar_id).execute()
print(result['items'][0])
(I’m sorry I can’t take a screenshot of this one since it has sensitive data on it but I’m sure you’ll get it and if not by any chance, leave a comment I’ll follow up)
Here comes the interesting part: I’m trying to create a method called create_event to create Google Calendar Events:
def create_event(start_time_str, summary, duration=1,attendees=None, description=None, location=None):
- Now I want you to go here and read about Calendar Event insertion reference, Simply scroll down to code section which includes Python code sample and that’ll do.
- Second thing is, Quickly read through the library datefinder which allows us to simply enter a date as a string.
- Data Pretty Printer for a nice firm output in Command line/ Terminal
Now this is how my function looks like at the end:
def create_event(start_time_str, summary, duration=1,attendees=None, description=None, location=None):
matches = list(datefinder.find_dates(start_time_str))
if len(matches):
start_time = matches[0]
end_time = start_time + timedelta(hours=duration)
event = {
'summary': summary,
'location': location,
'description': description,
'start': {
'dateTime': start_time.strftime("%Y-%m-%dT%H:%M:%S"),
'timeZone': timezone,
},
'end': {
'dateTime': end_time.strftime("%Y-%m-%dT%H:%M:%S"),
'timeZone': timezone,
},
'attendees': [
{'email':attendees },
],
'reminders': {
'useDefault': False,
'overrides': [
{'method': 'email', 'minutes': 24 * 60},
{'method': 'popup', 'minutes': 10},
],
},
}
pp.pprint('''*** %r event added:
With: %s
Start: %s
End: %s''' % (summary.encode('utf-8'),
attendees,start_time, end_time))
return service.events().insert(calendarId='primary', body=event,sendNotifications=True).execute()
Now I’m going to call this method:
create_event(’24 Jul 12.30pm’, “Test Meeting using CreateFunction Method”,0.5,”dkalpa@email.com.au”,”Test Description”,”Mentone, VIC,Australia”)
Let’s go to our command prompt and execute our python script:
PS: Before doing that, Check this for the full code and double check that all the cutleries are there for the feast.

Now, Let’s go to Google Calendar and see if this one actually created or not!
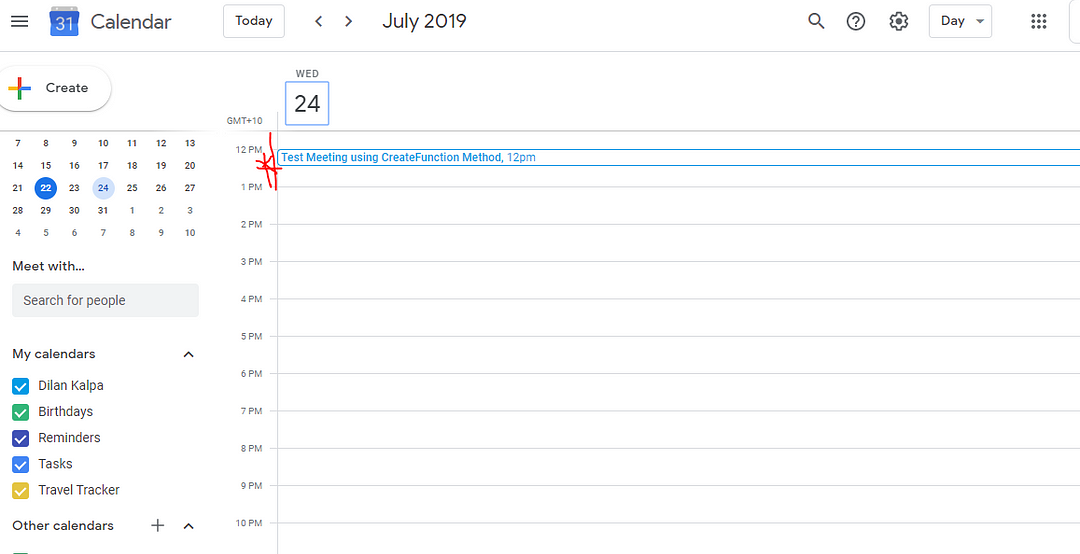
Thank you for reading the article till the end, I had to cut a lot of detail description that’s why inserted a bunch of reference links but if you run into any kind of errors or bugs please let me know. Meanwhile, Check out my article on How to Access Google Spreadsheets from Python because what’s really interesting is that, Imagine if you can create a spreadsheet including all the details of upcoming meetings, and use both Google Spreadsheet API and Calendar API to combine them together so we can create more than one event using our spreadsheet to our calendar, It’s basically a combination of this article and the above-mentioned one.
Imagine if you have a spreadsheet including all the details of upcoming meetings, and use both Google Spreadsheet API and Calendar API to combine them together so we can create more than one event using our spreadsheet to our calendar. Cool right?
Yes, So I’m working on it and will publish that article soon ;)
Till then, Adios!
Keen on getting to know me and my work? Click here for more!
Like all great stories, Let's start with the History. 1980's to early 1990's-Focus on computerizing business…www.jayasekara.blog
0 Comments